Note: Sample test is available on GitHub. See ChromeDragDrop folder.
Create a new Cross-browser test in Rapise and choose Chrome HTML web profile when prompted. Open your Google Drive folder in Chrome.
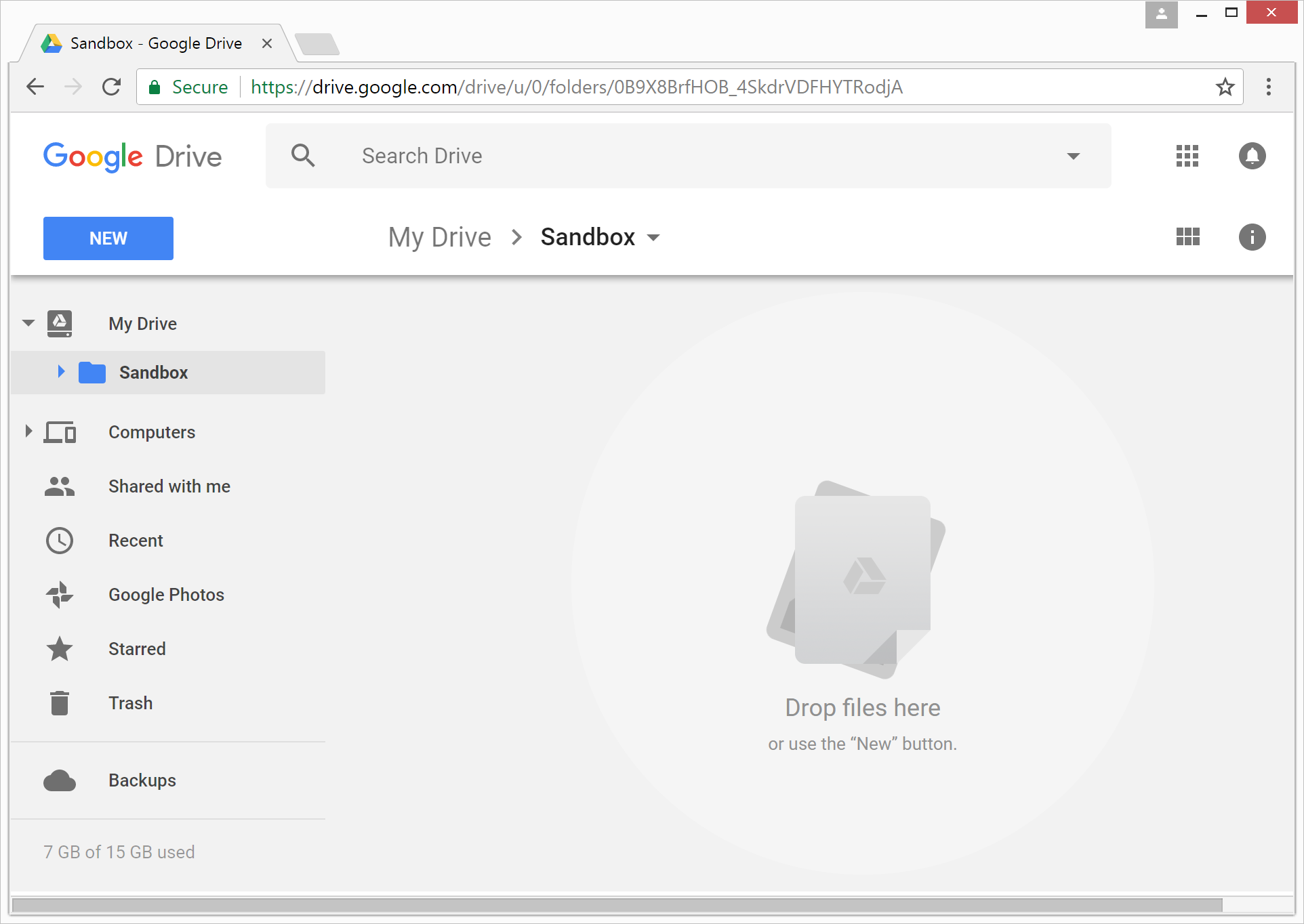
Start recording in Rapise and learn 'Drop files here' label. Finish recording and update object XPATH in properties window to
//div[@role='main' and not(@style)]
This is one of the most reliable ways to identify the drop target. DOM tree of the Google Drive is pretty complex, so construction of such an XPATH is pretty much a challenge for beginners. Most of DOM elements are always present on a page though sometimes stay invisible. And sometimes there are several copies of a given DOM sub tree.
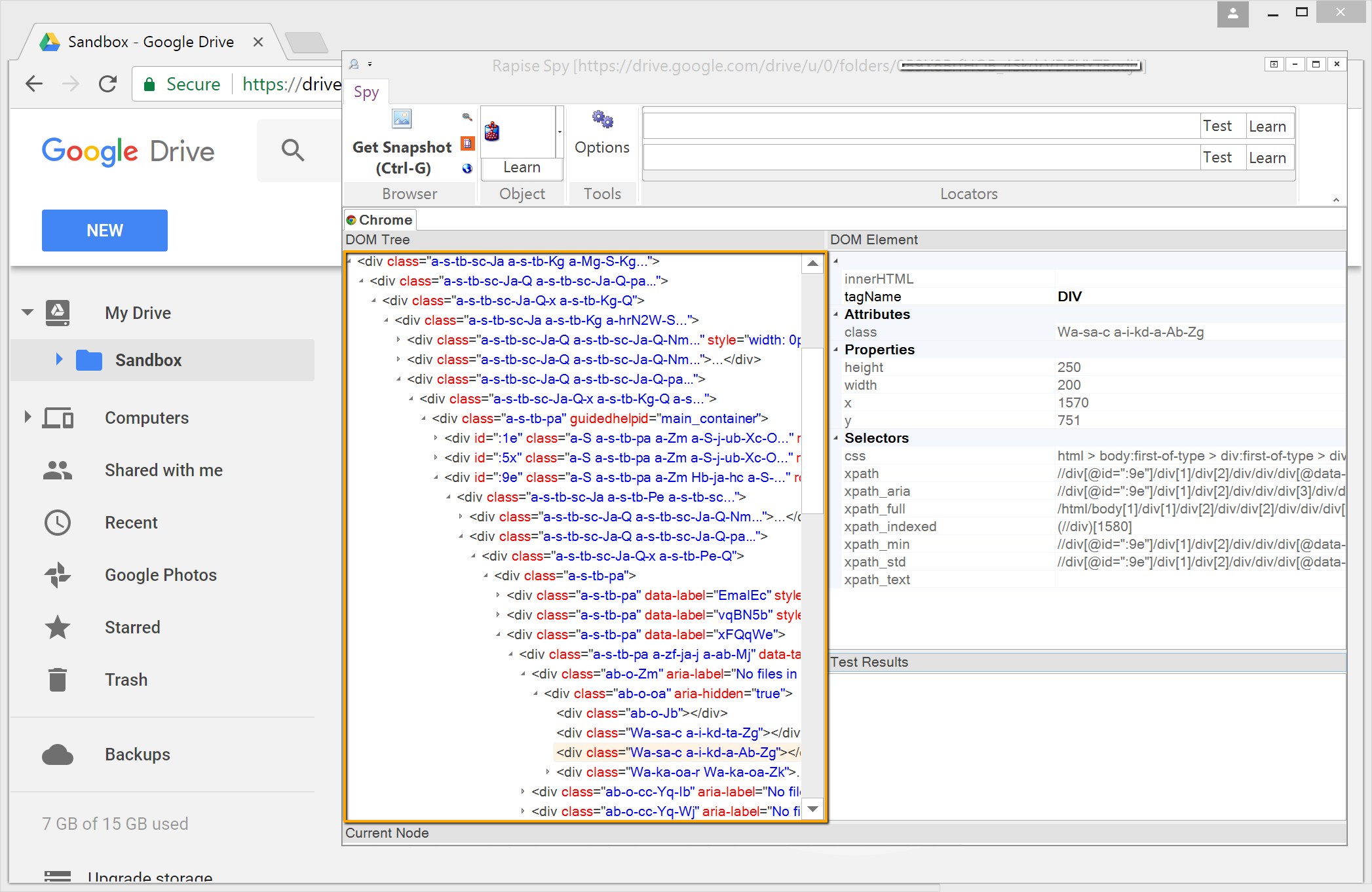
Ok, we finished with browser recording part. Let's proceed to Windows Explorer. Add 'UIAutomation' library to the list in the main test file.
g_load_libraries=["%g_browserLibrary:Chrome HTML%", "UIAutomation"];
Open Windows Explorer in a folder with files and start recording in Rapise. Learn the list of files.
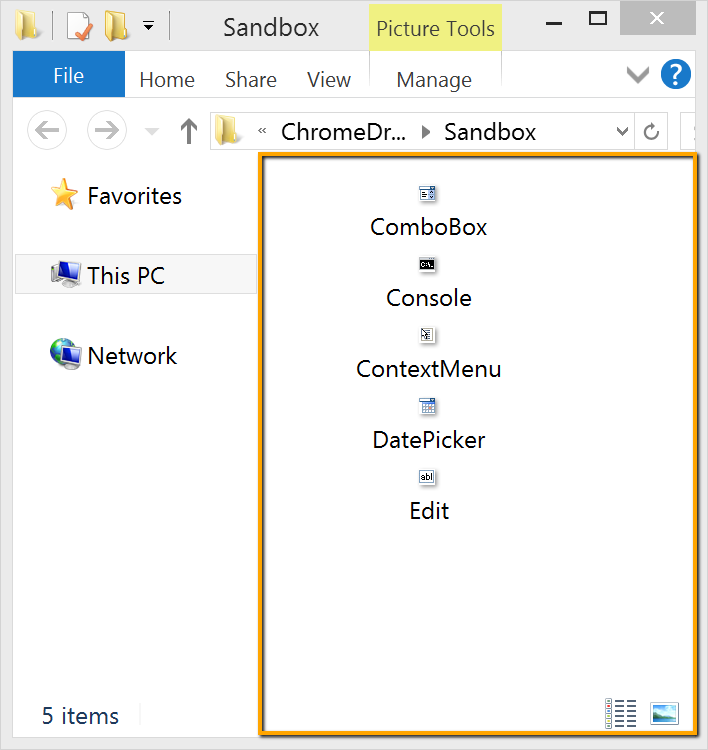
We are ready for drag&drop implementation. Here is the code. 'UploadArea' is a drop area in Chrome browser. 'Items_View' is the list of files in Windows Explorer. In the test we iterate through all files in the folder and drag&drop them into Chrome window. 'dropX' and 'dropY' designate the center of the drop area in Google Drive.
function Test()
{
/** target */
var target = SeS('UploadArea');
var dropX = target.GetX() + (target.GetWidth() >> 1);
var dropY = target.GetY() + (target.GetHeight() >> 1);
Tester.Message("dropX: " + dropX + ", dropY: " + dropY);
/** source */
var fileList = SeS('Items_View');
var itemCount = fileList.GetItemCount();
for(var i = 0; i < itemCount; i++)
{
var itemName = fileList.GetItemNameByIndex(i);
var source = fileList.DoFindByText(itemName);
/** drag & drop */
// move mouse to the center of the source object
source.DoMouseMove();
// press left mouse button
source.DoLButtonDown();
// drag the object over the drop area,
// give Chrome a chance to understand it is a dragover event
Global.DoMouseMove(dropX - 200, dropY - 50);
Global.DoSleep(300);
Global.DoMouseMove(dropX - 100, dropY);
Global.DoSleep(300);
// release mouse button
source.DoLButtonUp();
// pause before next drag&drop
Global.DoSleep(200);
}
// Let video capture to do the job
Global.DoSleep(5000);
}
Watch playback session of the test on YouTube