Problem
You try to record a web test for a Fultter application and interaction with any element is recorded as click on a canvas element.
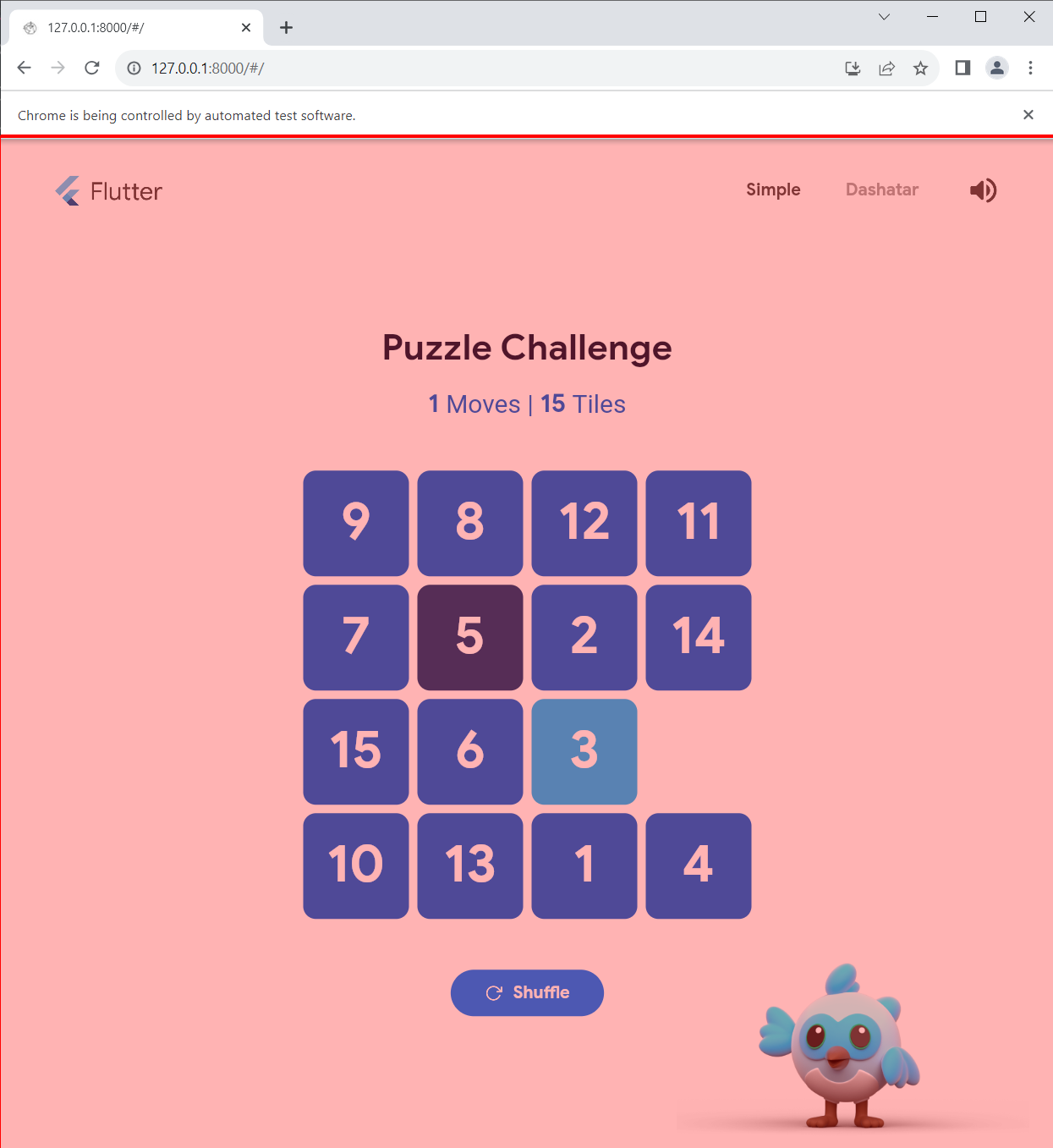
We are using Slide Puzzle sample application as an example.
https://github.com/VGVentures/slide_puzzle
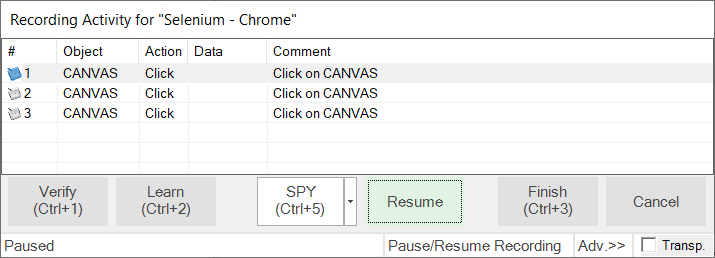
The Web Spy shows that the canvas element has no children.

Solution
Add the following function to your test.
function FlutterEnableAccessibility()
{
Navigator.DoWaitFor("//flt-glass-pane", 30000);
var xpath = "//flt-glass-pane@#@css=flt-semantics-placeholder";
var obj = Navigator.DoWaitFor(xpath, 5000);
if (obj)
{
obj.object_name = "EnableAccessibility";
obj.DoClick();
}
}
This function finds the 1x1 pixel size hidden button flt-semantics-placeholder and clicks it. As a result the DOM tree is populated with UI elements and you get an opportunity to record/play tests.
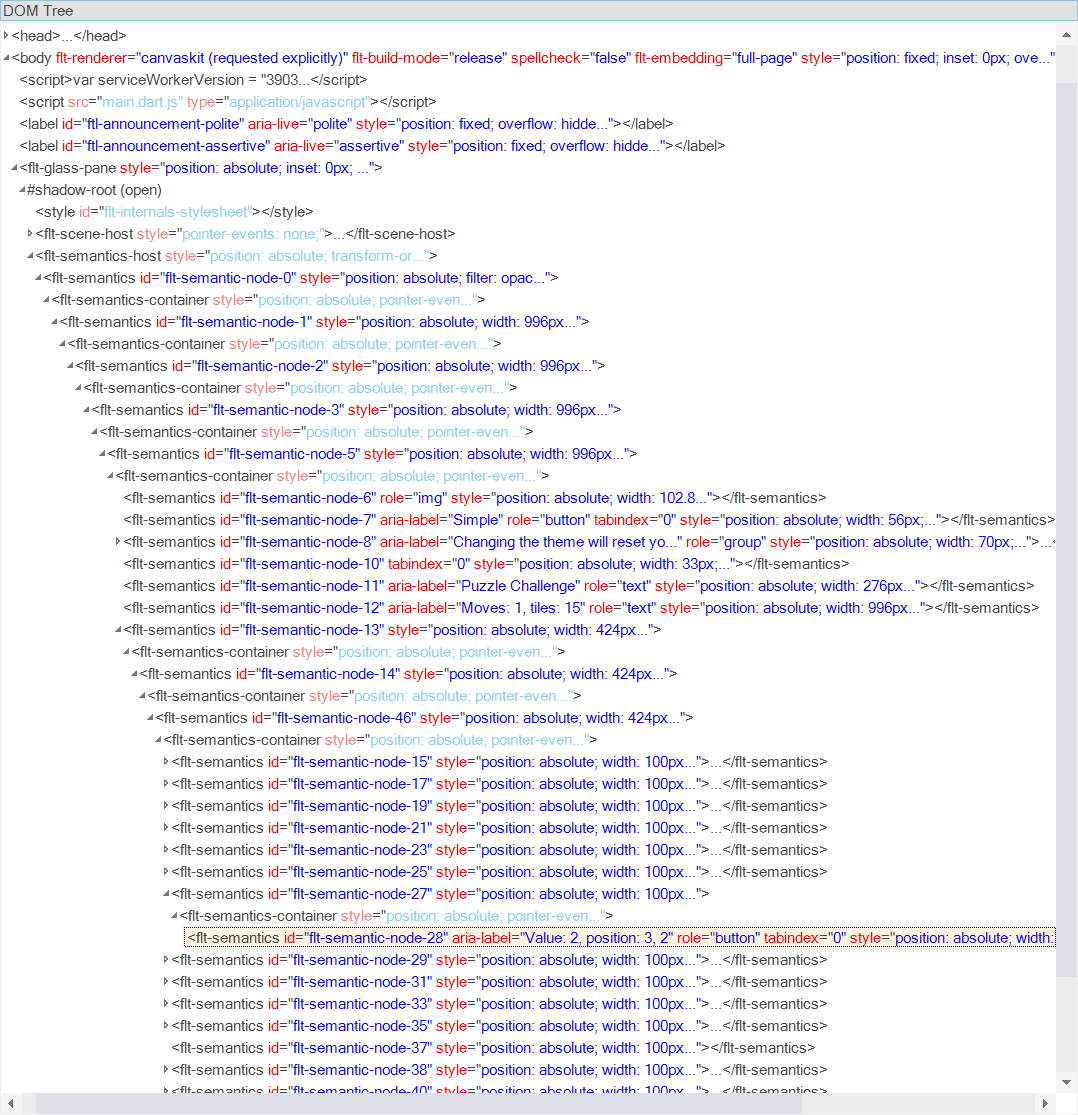
The easiest way to run FlutterEnableAccessibility function is to call it from RVL:

Important Notes
Since Flutter UI elements are placed inside Shadow DOM under flt-glass-pane element you will likely need to use the Web Spy to refine selectors and learn elements. Default recorded selectors are not very good since they are inside Shadow DOM.
Compare default recorded:
/html/body/flt-glass-pane@#@css=flt-semantics-host > flt-semantics > flt-semantics-container > flt-semantics > flt-semantics-container > flt-semantics > flt-semantics-container > flt-semantics:nth-of-type(2) > flt-semantics-container > flt-semantics > flt-semantics-container > flt-semantics:nth-of-type(5) > input
with manually written
//flt-glass-pane@#@css=input[aria-label='Enter your User ID']
Or another example. Recorded:
/html/body/flt-glass-pane@#@css=flt-semantics-host > flt-semantics > flt-semantics-container > flt-semantics > flt-semantics-container > flt-semantics > flt-semantics-container > flt-semantics > flt-semantics-container > flt-semantics:first-of-type > flt-semantics-container > flt-semantics:nth-of-type(7) > flt-semantics-container > flt-semantics > flt-semantics-container > flt-semantics > flt-semantics-container > flt-semantics > flt-semantics-container > flt-semantics
Manually written:
//flt-glass-pane@#@css=flt-semantics[aria-label*='Value: 3,']
Learn mode about Shadow DOM in Rapise:
https://rapisedoc.inflectra.com/Guide/selenium_webdriver/#shadow-dom
Sample Framework
Please find a sample framework attached (requires Rapise 8.0+). It has a Module named Flutter with action EnableAccessibility (alternative way of defining FlutterEnableAccessibility function). This action must be executed once for a loaded application to enable recording/playback.

Download sample framework
CSS Tricks
Finding elements in a Flutter application may be tricky.
Find CSS basics here:
https://devhints.io/css
Learn pseudo-classes:
https://developer.mozilla.org/en-US/docs/Web/CSS/Pseudo-classes
:has() pseudo-class is pretty handy
https://developer.mozilla.org/en-US/docs/Web/CSS/:has
Use Chrome DevTools to test selectors in real-time:
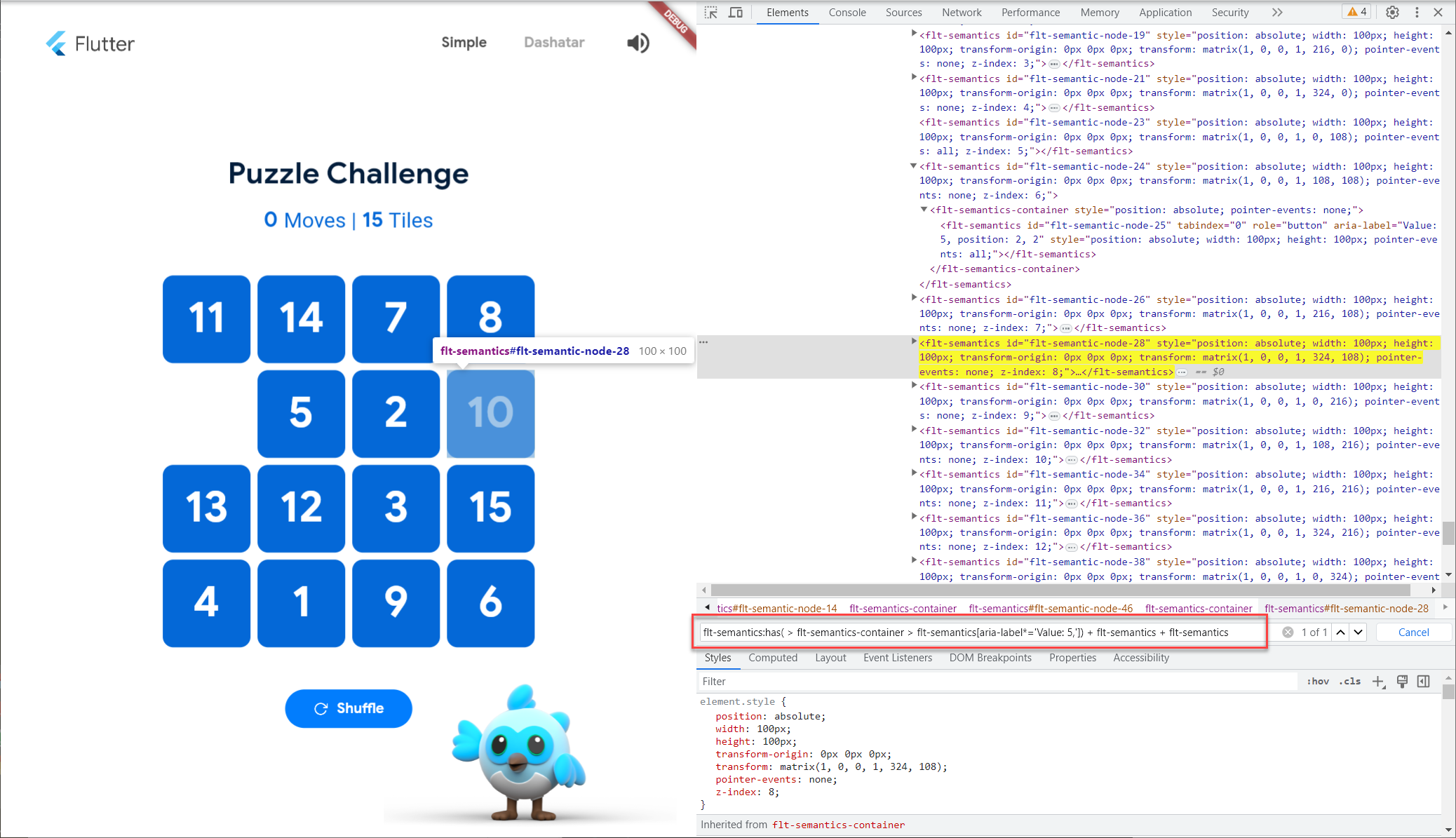
Ctrl+F opens the search bar (highlighted).